I Picked up a couple of round 240*240 pixel OLED display modules, as luck would have it they scale easily into the InMoov face frame-work.
Driving the display is an ESP32 using SPI bus for control... meaning that two displays can be controlled with it.
It uses the #include <Arduino_GFX_Library.h>
Here is the stripped down code for a simple generic ESP32 Eye |
#define JPEG_FILENAME "/240test.jpg" #include <Arduino_GFX_Library.h> #define TFT_CS 17 #define TFT_DC 16 #define TFT_RST 4 Arduino_DataBus *bus = new Arduino_ESP32SPI(TFT_DC, TFT_CS, 18 /* SCK */, 23 /* MOSI */, -1 /* MISO */, VSPI /* spi_num */); Arduino_GC9A01 *gfx = new Arduino_GC9A01(bus, TFT_RST, 0 /* rotation */, true /* IPS */); #include <SPIFFS.h> #include "JpegClass.h" static JpegClass jpegClass; // pixel drawing callback static int jpegDrawCallback(JPEGDRAW *pDraw) { gfx->draw16bitBeRGBBitmap(pDraw->x, pDraw->y, pDraw->pPixels, pDraw->iWidth, pDraw->iHeight); return 1; } void setup() #ifdef TFT_BL if (!SPIFFS.begin()) // read JPEG file header void loop() |
The library supports BMP,Jpeg.....
;Png,Mjpeg and even .....
gifs.
The examples above uses the "SPIFFS" file system.
It is a simple case of uploading your program, then uploading the graphics into the ESP's SPIFF file system.
If you have not used the SPIFFS system on your ESP32 before then it is essential to install the driver for it into your Arduino IDE, you only need to do this once. Tutorial Here :- Randomnerds
Alternate HTTP Server (aka local access) |
#define JPEG_FILENAME1 "/blueeye.jpg" #define JPEG_FILENAME2 "/browneye.jpg" #define JPEG_FILENAME3 "/darkbrowneye.jpg" #include <Arduino_GFX_Library.h> #define TFT_CS 17 #define TFT_DC 16 #define TFT_RST 4 Arduino_DataBus *bus = new Arduino_ESP32SPI(TFT_DC, TFT_CS, 18 /* SCK */, 23 /* MOSI */, -1 /* MISO */, VSPI /* spi_num */); Arduino_GC9A01 *gfx = new Arduino_GC9A01(bus, TFT_RST, 0 /* rotation */, true /* IPS */); #include <SPIFFS.h> #include "JpegClass.h" static JpegClass jpegClass; // pixel drawing callback static int jpegDrawCallback(JPEGDRAW *pDraw) { gfx->draw16bitBeRGBBitmap(pDraw->x, pDraw->y, pDraw->pPixels, pDraw->iWidth, pDraw->iHeight); return 1; } #include <WiFi.h> const char* ssid = "Wifiname"; WiFiServer server(80); void setup() while (WiFi.status() != WL_CONNECTED) { // Init Display #ifdef TFT_BL if (!SPIFFS.begin()) { } void loop() if (client) { // if you get a client, // if the current line is blank, you got two newline characters in a row. // the content of the HTTP response follows the header: // Check to see if the client request was "GET /H" or "GET /L": |
Simple Two eye display driver code ( needing only one ESP32) |
#define JPEG_FILENAME1 "/blueeye.jpg" #define JPEG_FILENAME2 "/browneye.jpg" #include <Arduino_GFX_Library.h> #define TFT_DC 16 #define TFT_SCL 18 #define TFT_MOSI 23 #define TFT_MISO -1 #define TFT_CS1 17 // Display 1 Arduino_DataBus *bus1 = new Arduino_ESP32SPI(TFT_DC, TFT_CS1, TFT_SCL, TFT_MOSI, TFT_MISO , VSPI /* spi_num */); #include <SPIFFS.h> } if (!SPIFFS.begin()) { void loop() |
Now its time to refine the graphics , make a dual OLED holder and re-test.
The advantage of an Interactive system is that the display could also be used for Debug (during start up) or also feedback for control actions.....
Any other ideas out there ?.... note below and we see what can be done....
Oh my gosh ! ... So cool, and
Oh my gosh ! ... So cool, and yes - so much potential for displaying all sorts of info...
Heh I can imagine the veins increasing with red ...
then a red text dump of errors :D
I'm curious if you've thought of the communication to/from the esp32 ?
will you be doing websockets ?
I've been working quite a lot on Mqtt and MqttBroker service in MRL lately...
My house sensors are beginning to utilize mrl for communication configuration and coordiantion.
I have sensors which currently publish to Mrl's MqttBroker,
and can easilly imagine 2 way communication with your esp32 eyes.
publish blink message to topic /eyes/blink and blink go the eyes

publish text message to /eyes/text .. and it displays text
many possiblities ! (I'm really starting to like mqtt)
Update HTTP Jpeg & gifs
Framework works.... now finicky work on the graphics....
Intergrated the Gif and JpegClass's (shoehorn time).
Here's a sneak peak of the
Here's a sneak peak of the new MqttBroker service in MRL
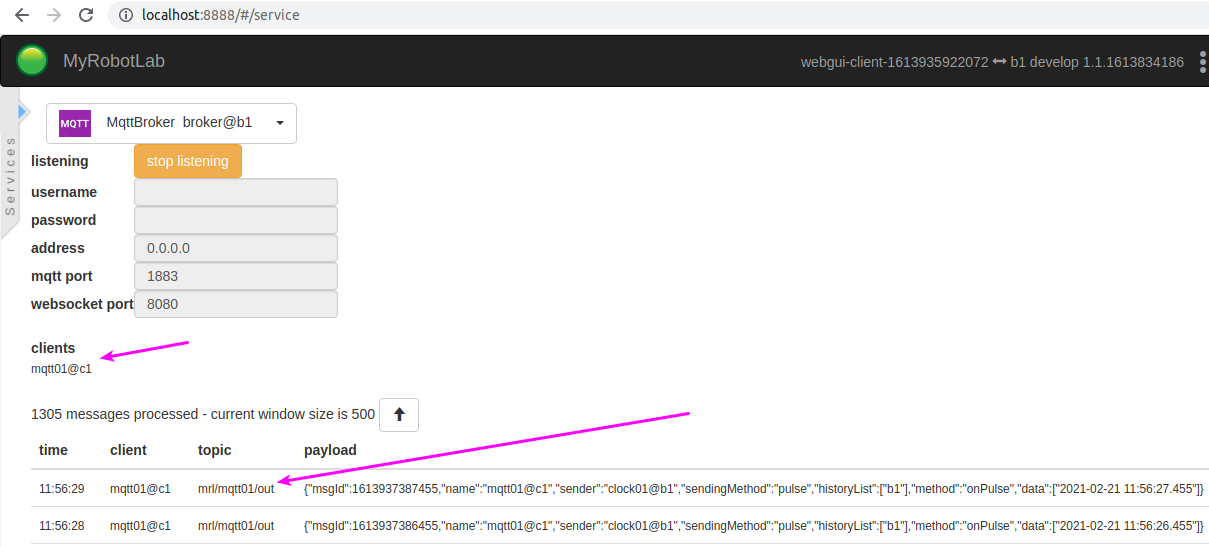
Your esp32 would connect to mrl, and subscribe to /rightEye/blink topic perhaps then from MRL or potentially any device that can send mqtt messages - you'd be winking ;)
HTTP Server ala "local"
I tend to keep things local... so current setup is an ESP32 HTTP server, however in this format anything is possible I guess.
Broker !!
I looked into MQTT .... however could only find cloud service (off site) brokers....
Are Cload brokers the only way to go or are there local brokers that can be instanced?
edit :- did I miss something.... does MRL act as a local broker...?
.... G digs deeper......
You got it .. Yeah, I've
You got it ..
Yeah, I've been working on it for a while ...
Ya Mrl can now "be a" broker - implementation is done, and I have a worky UI(s) too
Need to do some documentation now.
Mqtt Brokers I think are really quite good for orchestrating lots and lots of little IoT devices...
HTTP is nice from a browser - but its very synchronous ..
e.g. send a request ----> get a response back
With Mqtt Brokers anything can talk to anything potentially at any time...
You use "topics" which are names - and you send messages to them ..
Anything can subscribe to the topic and anything can send a message to that topic which will be broadcasted to all the subscribers ...
Sweet
Nice job, looks awesome!
X Y movement
Hello
This looks great.
I am working on the same idea using the animated_eyes_2 code for the ESP32 TFT_eSPI library.
How can I change the x y for the eyes on the arduino from servo's to analog voltage?
Could MTTQ be used to send the x y coordinates?
Cheers Richard