I recently ordered a Lidar Lite from Sparkfun... This is a very capable distance measurement device, a laser rangefinder in a very compact form and is also relatively cost effective... Pulsed Light company first carried out a successful Kickstarter last year and now the device is commercially available... Link to manufacturer: http://pulsedlight3d.com/
After playing a little, I managed to get this tiny device working... the setup can be seen in above pic... I placed the Lidar on a pan-tilt driven by an Arduino and sent distance measurement to PC on which the output is visualised by a Processing sketch... Arduino sketch:
/* Sweep Lidar Scanner* Lidar Lite sensor sending cm distance while servos sweep* Dincer Hepguler 2015*/#include <Servo.h>#include <I2C.h>#define LIDARLite_ADDRESS 0x62 // Default I2C Address of LIDAR-Lite.#define RegisterMeasure 0x00 // Register to write to initiate ranging.#define MeasureValue 0x04 // Value to initiate ranging.#define RegisterHighLowB 0x8f // Register to get both High and Low bytes in 1 call.Servo myXservo; // create servo object to control X servoServo myYservo; // create servo object to control Y servoint posX = 0; // variable to store X servo positionint posY = 0; // variable to store Y servo positionvoid setup(){Serial.begin(57600);myXservo.attach(10); // attaches X servo on pin 10 to the servo objectmyYservo.attach(9); // attaches Y servo on pin 9 to the servo objectI2c.begin(); // Opens & joins the irc bus as masterdelay(100); // Waits to make sure everything is powered up before sending or receiving dataI2c.timeOut(50); // Sets a timeout to ensure no locking up of sketch if I2C communication fails}void loop(){while (millis() > 30000);{for(posX = 30; posX <= 150; posX += 3) // goes from 30 degrees to 150 degrees{for(posY = 60; posY <= 140; posY += 2){ //Y axis sweepmyXservo.write(posX); // tell servo to go to position in variable 'pos'myYservo.write(posY);//delay(1); // waits for the servo to reach the positionGetDist();}for(posY = 140; posY >=60; posY -=2){ //Y axis sweepmyXservo.write(posX); // tell servo to go to position in variable 'pos'myYservo.write(posY);//delay(1); // waits for the servo to reach the positionGetDist();}}for(posX = 150; posX >=30; posX -=3) // goes from 150 degrees to 30 degrees{for(posY = 60; posY <= 140; posY += 2){ //Y axis sweepmyXservo.write(posX); // tell servo to go to position in variable 'pos'myYservo.write(posY);//delay(1); // waits for the servo to reach the positionGetDist();}for(posY = 140; posY >=60; posY -=2){ //Y axis sweepmyXservo.write(posX); // tell servo to go to position in variable 'pos'myYservo.write(posY);//delay(1); // waits for the servo to reach the positionGetDist();}}}myXservo.write(90);myYservo.write(90);delay(25);}void GetDist(){// Write 0x04 to register 0x00uint8_t nackack = 100; // Setup variable to hold ACK/NACK resopnseswhile (nackack != 0){ // While NACK keep going (i.e. continue polling until sucess message (ACK) is received )nackack = I2c.write(LIDARLite_ADDRESS,RegisterMeasure, MeasureValue); // Write 0x04 to 0x00delay(1); // Wait 1 ms to prevent overpolling}byte distanceArray[2]; // array to store distance bytes from read function// Read 2byte distance from register 0x8fnackack = 100; // Setup variable to hold ACK/NACK resopnseswhile (nackack != 0){ // While NACK keep going (i.e. continue polling until sucess message (ACK) is received )nackack = I2c.read(LIDARLite_ADDRESS,RegisterHighLowB, 2, distanceArray); // Read 2 Bytes from LIDAR-Lite Address and store in arraydelay(1); // Wait 1 ms to prevent overpolling}int distance = (distanceArray[0] << 8) + distanceArray[1]; // Shift high byte [0] 8 to the left and add low byte [1] to create 16-bit int// Print Distance and axis posSerial.print(posX);Serial.print(",");Serial.print(posY);Serial.print(",");Serial.println(distance);}
Sorry for poor pic quality.. The Processing sketch is on the pic... It creates a visual image of point cloud created by Lidar...
The pixel color shows depth, like Kinect, the darker the pixel, the closer the object is...


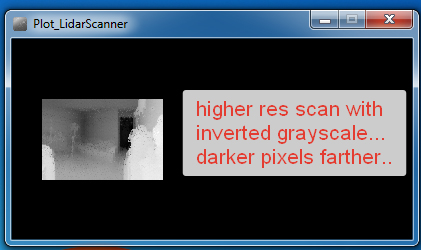
The cloud image is tiny so pls zoom in to see detail... The results are promising... I loved this tiny gadget...
Update:
I tried to reach a max scan speed and achieved 6 seconds front scan speed with Arduino. This is the maximum because either the servos are misbehaving or the sensor is misreading beyond that. So I will now try to implement a rotating 360degree scan with either Raspberry Pi or Omega2...
Awesome project!
Hi!
My name is Bernhard and I run a forum at www.diy3dscan.com at which we collect all diy 3d scan projects. I made a post about your project. I would be very happy, if you join the forum and tell us more about this amazing project.
Best,
Bernhard
www.virtumake.com
Lidar 3D scan...
Thx Bernard,
Actually I made this DIY Lidar Scan to make some sort of use about it for my robot projects... However I am still trying to find a way to implement 3d virtualization out of it... If I can manage to make an 3D virtual image out of this depth map I will be pleased... Still short of ideas...
Dincer
How are you creating the Point Cloud?
Curious to how you are creating a pont cloud on this device, it doesn't look like it's been modified.
Lidar Lite Point Cloud...
It is not modified... It is on a pan-tilt which sweeps the area in 1 degree angles in X and Y axes...Lidar takes fast measurements during moving and Point Cloud is generated this way...
Dincer
Meshing Pointclouds
You can use your 3d data to generate a polygon mesh with the software Meshlab, or you could try to reverse-engineer your room based on the pointcloud data with Sketchup.
Code for PlotServoScan
Can you post the complete code for plotting the scan data and creating the point cloud image? It looks like most of it is showing in the window, but I'm not sure if it is completely visible. Also. I use the Arduino IDE and have never used Processing. Can it be run in the Arduino IDE or do I need to install Processing to get the plot window?
Lidar Scanner...
You have to install Processing in order to create the plot... Arduino and Lidar sends the data and Processing makes the visualization of depth map..
What are the exact steps
Hi there,
What are the exact steps needed to get this to work, and what exactly is needed software wise to get an image to show?
Thanks,
Kevin S. Chambers
Is all the code there?
I'd also like to try doing this, curious if all the code is there? As well curious about the sketch function, is it an Arduino library?
That look really cool. I am
That look really cool. I am Impressed how about how great the depth image looks.
What pan tilt drive are you using? and do you have any idea of the angular precision and accuarcy?.
how did you get the colors
how did you get the colors inversed?
lidar z map...
Hi,
What colors?... this ia not a camera image... it is built as a z map.. from Lidar measurements over the area... It is grayscale 0-255 indicating depth from measuring point... regards...
Dincer
lidar z map...
hi,
I used a simple plastic pan-tilt with 9g servos as can be seen in the pic... angular precision accuracy is 1 degrees per axis... regards..
Dincer
lidar scanner
Hi Alan,
I use the uploaded sketch in above post and I have not seen any problems with it.. It completes the scan everytime I attempted in any room..
regards...
Dincer
I2C Library
I have one of these sensors and they are wonderful but if you use the Wire library they can sometimes freeze.
What I2C library are you using? It looks like it might be the I2C Master Library from
dsscircuits.com/articles/86-articles/66-arduino-i2c-master-library
Unfortunately I cannot get it to work which I think is due to the use of repeated starts that the Lidar Lite cannot handle. Have you met these problems and how have you handled them.
Alan
I2C Library
If you are not having problems then it is my understanding that you are in a minority of one - which makes my interest in which I2C library you are using even more keen. Can you direct me to where I can get a copy of the library you are using?
Alan
lidar lite
Alan,
Have you visited Pulsed light website and read everything about Lidar Lite?... There are docs about using Lidar Lite and many examples there... I developed my script using the basics from there... The I2C.h lib for the Lidar Lite is also mentioned there... For your convenience the link is: http://www.dsscircuits.com/index.php/articles/66-arduino-i2c-master-lib…
and the link for the Lidar Lite docs:
http://lidarlite.com/docs/v2/
Pls take a look... you can use my script given in my above post for trial... regards...
Dincer
I2C Library
As far as I can see all the the Pulsed light examples and their library use the Wire library for I2c comms.
The problem with that library is that it is full of while loops and if a response is missed for whatever reason then it can freeze.
The I2C master library gets round that problem but it also uses repeated starts that the Lidar Lite cannot handle.
That is why I am so interested in your library.
I have tried your code with the servo bits removed and I get the exact same result as always witthe that library - all reads return zero (and no errors).
I was hoping that perhaps you had an old version of the library as there has been some relatively recent rewrite.
Alan
Lidar Lite....
Alan,
I used the "LidarLiteI2ClibraryGetDistanceContinuousRead" example (not PWM) as a base for my script... It is under "LidarLiteBasicsMaster" examples... If you think my copy of I2C lib is different I can post my copy here.... But unfortunately I donot know how to attach file here... :)
I2c Library
I cannot think of any other solution but that you have a different version of the Library.
If you have DropBox you could put acopy in your public folder and publish the link.
One other thought. It looks from the picture that you may have the silvered labelled version of the sensor (version 1)
I have the blue labelled version so that might be part of the difference although I understand that the silver label version had even more problems with the I2C comms.
Alan
hi, I am currently try to
hi,
I am currently try to create a simple distance measurement prototype by using Lidat lite v3. But I have to use pic16f877a microcontroller with i2c interface. I couldn't make my lidar work and have been stuck for a long time. Can anyone help me please? There are not much examples I can look up to. I couldn't attach my codes here. Please kindly let me know if anyone can help with source code and any suggestions.
Thank you
MM