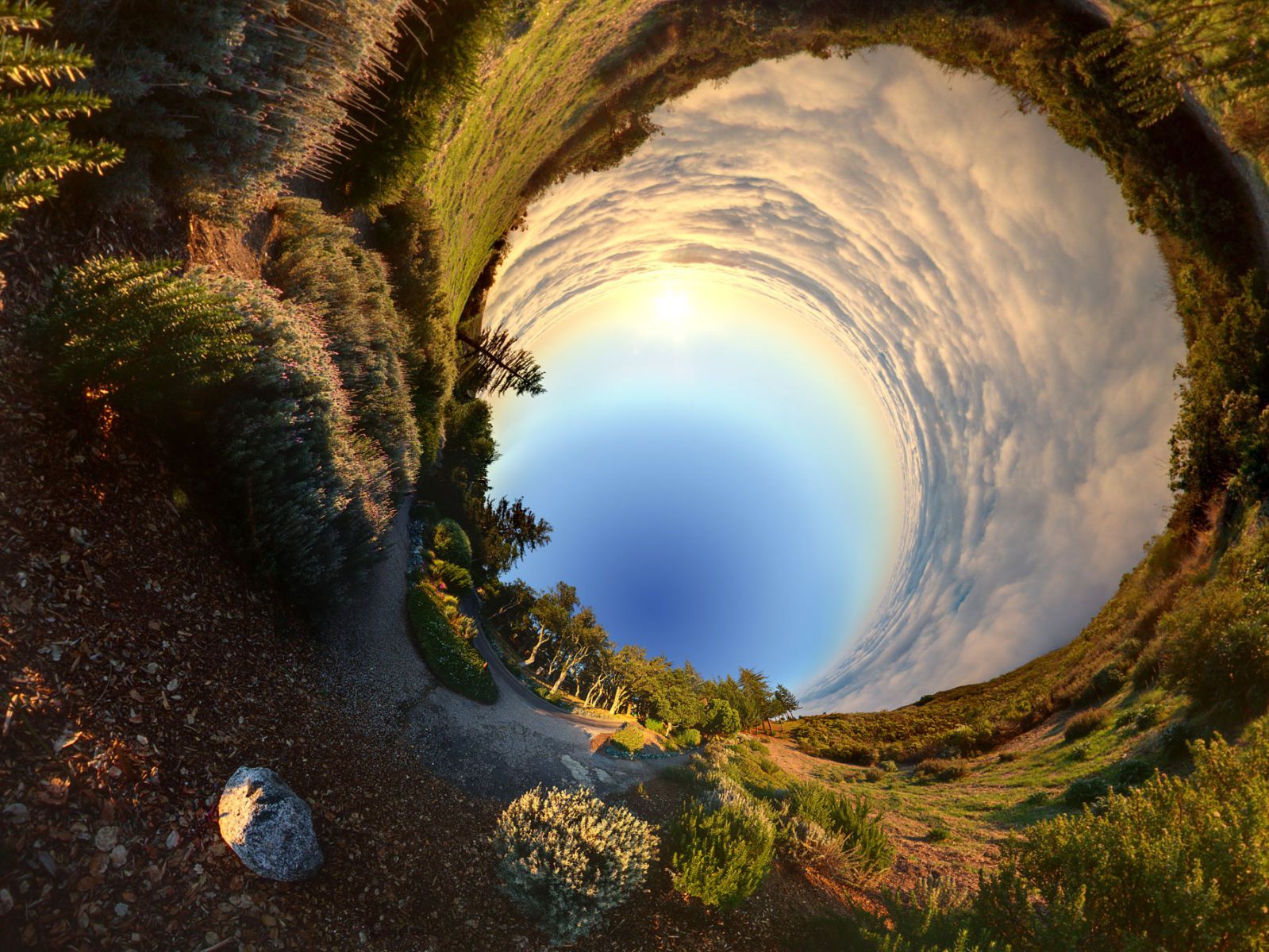
Setting the arduino board type needs to come from the user's UI or helper functions like arduino.setBoardUno().
Currently the board can set the type, by data coming back from the board. Although I would prefer this method, if Arduino & its firmware was capable of providing all the necessary information. But its not. Sad, too, because I like "plug and play"
If we choose to support upload - I think we have to support sending the correct processor type (especially if its not atmega328, which 'might' be default for the compiler.
I've added a new method to Arduino.getBoardTypes() - this returns a list of board types. Each BoardType contains a "name","board" and "id" - Name is what is displayed, and board is a unique string key, and id is a unique integer.
I'll be adding getBoardTypes() to Microcontroller interface - all microcontrollers should be able to easily implment this.
The next part is using the BoardType.board to create the PinDefinitions
I recently added the static :
PinDefinitions Microcontroller.createPinDefintions(String boardType)
Which means you can build a list of PinDefinitions based on a board type without having to create an instance of the board ( ... goodtimes ..)
All of this should handle all Arduino boards and is generic enough to handle all the Microcontrollers I can think of..
Now I've got to figure out which PinDefinitions belong with which Arduino boardTypes ..
If your curious .. this is the BoardTypes parsed from boards.txt. If a dot is in "board" - the upload command will be
args.add("arduino:avr:" + parts[0] + ":cpu=" + parts[1]);
otherwise
args.add("arduino:avr:" + parts[0]);
(I think this will handle all cases)
"boardTypes": [
{
"name": "LilyPad Arduino USB",
"board": "LilyPadUSB",
"id": -1212586885
},
{
"name": "Arduino NG or older - atmega168",
"board": "atmegang.atmega168",
"id": 1518858014
},
{
"name": "Arduino NG or older - atmega8",
"board": "atmegang.atmega8",
"id": 417222499
},
{
"name": "Arduino BT - atmega168",
"board": "bt.atmega168",
"id": -956038446
},
{
"name": "Arduino BT - atmega328",
"board": "bt.atmega328",
"id": -956036648
},
{
"name": "Arduino Industrial 101",
"board": "chiwawa",
"id": 746594744
},
{
"name": "Adafruit Circuit Playground",
"board": "circuitplay32u4cat",
"id": 933188827
},
{
"name": "Arduino Duemilanove or Diecimila - atmega168",
"board": "diecimila.atmega168",
"id": -436756937
},
{
"name": "Arduino Duemilanove or Diecimila - atmega328",
"board": "diecimila.atmega328",
"id": -436755139
},
{
"name": "Arduino Esplora",
"board": "esplora",
"id": -1452294284
},
{
"name": "Arduino Ethernet",
"board": "ethernet",
"id": -1419358249
},
{
"name": "Arduino Fio",
"board": "fio",
"id": 101388
},
{
"name": "Arduino Gemma",
"board": "gemma",
"id": 98239779
},
{
"name": "Arduino Leonardo",
"board": "leonardo",
"id": 1970939284
},
{
"name": "Arduino Leonardo ETH",
"board": "leonardoeth",
"id": -245593211
},
{
"name": "LilyPad Arduino - atmega168",
"board": "lilypad.atmega168",
"id": 20763209
},
{
"name": "LilyPad Arduino - atmega328",
"board": "lilypad.atmega328",
"id": 20765007
},
{
"name": "Arduino/Genuino Mega or Mega 2560 - atmega1280",
"board": "mega.atmega1280",
"id": -1899434886
},
{
"name": "Arduino/Genuino Mega or Mega 2560 - atmega2560",
"board": "mega.atmega2560",
"id": -1899402274
},
{
"name": "Arduino Mega ADK",
"board": "megaADK",
"id": 943274710
},
{
"name": "Arduino/Genuino Micro",
"board": "micro",
"id": 103890628
},
{
"name": "Arduino Mini - atmega168",
"board": "mini.atmega168",
"id": -1129277289
},
{
"name": "Arduino Mini - atmega328",
"board": "mini.atmega328",
"id": -1129275491
},
{
"name": "Arduino Nano - atmega168",
"board": "nano.atmega168",
"id": -1479385420
},
{
"name": "Arduino Nano - atmega328",
"board": "nano.atmega328",
"id": -1479383622
},
{
"name": "Linino One",
"board": "one",
"id": 110182
},
{
"name": "Arduino Pro or Pro Mini - 16MHzatmega168",
"board": "pro.16MHzatmega168",
"id": 2116770325
},
{
"name": "Arduino Pro or Pro Mini - 16MHzatmega328",
"board": "pro.16MHzatmega328",
"id": 2116772123
},
{
"name": "Arduino Pro or Pro Mini - 8MHzatmega168",
"board": "pro.8MHzatmega168",
"id": -1982008122
},
{
"name": "Arduino Pro or Pro Mini - 8MHzatmega328",
"board": "pro.8MHzatmega328",
"id": -1982006324
},
{
"name": "Arduino Robot Control",
"board": "robotControl",
"id": 2013718419
},
{
"name": "Arduino Robot Motor",
"board": "robotMotor",
"id": -1865756629
},
{
"name": "Arduino/Genuino Uno",
"board": "uno",
"id": 115958
},
{
"name": "Arduino Uno WiFi",
"board": "unowifi",
"id": -280884981
},
{
"name": "Arduino Yún",
"board": "yun",
"id": 120018
},
{
"name": "Arduino Yún Mini",
"board": "yunmini",
"id": -826654679
}
]
Great! Look like MRL gonna
Great!
Look like MRL gonna support all those Arduino boards. And if new board appear in the future, parsing again board.txt is probably all that will be required to have the new board working.
But what about the non-Arduino board, like ESP or teensy? I don't know much about the teensy, but MrlComm can work in the ESP board (with a few prepocessor modification)
We will certainly support the
We will certainly support the ESPs ..
I glanced at some of the #defines
I assume you manually set #define ESP8266 ?
We could have ArduinoUtils add a define depending on the BoardType.board
Additionally we coul modify the source - so that an int is set to the appropriate BoardType.id :P
However, if we decide to let MRL modify the source, it means the source will not be correct outside of compiling & uploading through MRL ... this is a possible "slippery slope"
the #define ESP8266 is set by
the #define ESP8266 is set by the ESP library, So no I did not manually set #define ESP8266. That define become set when you select any ESP board as board type.
I have never try to upload to an ESP board using the command line, but I expect the ESP8266 define will be set by passing the board type to the arduino ide, provide the ESP library is installed in the arduino ide library
Well that will be nice .. It
Well that will be nice ..
It means I just have to add another BoardType to the list created by boards.txt in getBoardTypes()
I have an ESP8266 which I'll test when I get there...
esp8266 boards manager
The Arduino Boards manager gets the esp8266 info from here:
http://arduino.esp8266.com/versions/2.3.0/package_esp8266com_index.json
I like that they have it in
I like that they have it in json, nicer than the old Arduino property files.
So, I'm guessing the selection process you select on "name" - and the tools, compilers etc specified in the file and in other downloades, are key'd by that name ?
For example if I choose, "Phoenix 2.0" what does that change ?
I parsed the Arduino boards.txt and created new BoardTypes for Arduinos which can have different processors and require different parameters - wondering what parameters the "Phoenix 2.0" requires..
I find Arduino's UI buggy in the sense you can select Nano - and the ui hides the fact you need to select which processor you have. This happend with my Arduino Duemilanove all the time - in MRLs upcoming list - each BoardType is in the select list, hopefully this will be less confusing..
Can the "Phoenix 2.0" be uploaded by the command line tool ? - if so what is the "board" for Phoenix 2.0 ? Does it not exist - or is it passed to the downloaded 'custom' compiler for ESP8266 ?
More to the point Mats, what do you use - and what do you need to select to make it work correctly ?
Board info
After you select the board, you can select a lot of different configurations.
So for example for the "Generic esp8266" you get choises for:
Flash Mode: ( DIO, QIU, DOUT, QOUT )
Flash frequency ( 40MHz, 80 Mhz)
CPU frequency ( 80Mhz, 160 Mhz )
Flash size ( 13 different options, where you can select how much memory the board has and how much should be used as an emulated SSD disk )
and several other parameters.
And even after selecting all the different parameters, there is no way to know what pin's ha been made avaliable on the board you use.
If I change to a different board like Phoenix 2.0 I get a different set of options.
You can see it in the Arduino IDE. Files => Preferences ==> Addition boards manager URL:s
Add the path http://arduino.esp8266.com/stable/package_esp8266com_index.json
Restart the Arduino IDE.
In Tools ==> Board: select the Board that you are interested in and see how the options change in the Tools list for each different board.
Looks like a deep rabbit hole to me.
Oh .. another bonus regarding
Oh .. another bonus regarding the static interface method
Microcontroller.getBoardTypes()
is that its a single point of reference - so all UIs (WebGui, SwingGui, JavaFxGui) can be using the same source of data, so potentially they will all be consistent - and the process of getting the info, will be consistent too.